Python Syllabus
Course Duration: 60 Hrs
- Introduction to Python
Python is an interpreted, high-level programming language known for its readability. Students will learn how to install Python and set up their development environment. - Variables and Data Types
Covers the basic types like integers, floats, strings, and booleans. Learners will understand how to assign variables and check their types using Python. - Input and Output Operations
Explains how to collect user input with input() and display information using print(). It also covers string formatting for better output display. - Operators in Python
Introduces arithmetic, logical, comparison, and bitwise operators. Students will practice using operators to build basic expressions. - Conditional Statements
Covers if, elif, and else for decision-making in programs. Real-life scenarios will demonstrate when and how to use these conditions. - Loops
Discusses the for and while loops for iteration, along with break and continue for control flow. Students will create loops to handle repetitive tasks. - Functions
Teaches how to define reusable code blocks using functions. This section covers parameters, return statements, and default arguments. - Recursion
Introduces recursive functions, where functions call themselves to solve smaller subproblems. Students will practice implementing recursion. - Lists
Covers list creation, slicing, and common methods like append, pop, and sort. Learners will understand how to manipulate list data effectively. - Tuples
Focuses on immutable sequences used for fixed data. Students will compare tuples with lists and learn tuple unpacking techniques. - Dictionaries
Introduces key-value pairs, dictionary methods, and how to loop through dictionaries. Learners will use dictionaries to store structured data. - Sets
Covers sets, which store unique elements. Students will practice using union, intersection, and other set operations. - String Manipulation
Explains string methods such as split(), join(), and slicing. Learners will work with text data and formatting techniques. - Exception Handling
Teaches how to use try, except, and finally to handle errors. Students will explore real-life scenarios to build error-free programs. - File Handling
Explores reading and writing files using open() and with statements. Learners will build small programs to process text files. - Classes and Objects
Introduces object-oriented programming by defining classes and creating objects. Students will understand how to represent real-world entities. - Inheritance and Encapsulation
Covers how classes inherit properties from other classes. Students will also learn encapsulation for data protection within classes. - Polymorphism
Demonstrates how functions and methods can behave differently based on input or objects. Real-life scenarios will show polymorphic behavior. - Lambda Functions
Teaches the use of anonymous functions for short, one-time tasks. Students will explore how lambda works with map(), filter(), and reduce(). - Iterators and Generators
Explains how to create iterators for sequential data and generators for lazy evaluation. Students will practice with loops and data streams. - Decorators and Context Managers
Covers decorators to modify functions and with statements for efficient resource management. Students will create custom decorators. - Regular Expressions (Regex)
Teaches pattern matching with the re module. Students will search, extract, and manipulate strings using regex patterns. - Working with Dates and Time
Covers the datetime module for manipulating date and time. Learners will create programs that handle time-based operations. - Introduction to Libraries
Introduces libraries such as numpy for arrays, pandas for dataframes, and matplotlib for visualizations. Basic operations are demonstrated. - Data Handling with Pandas
Explores DataFrames for structured data handling. Students will perform filtering, sorting, and grouping operations on datasets. - Data Visualization with Matplotlib
Teaches how to plot data using different types of charts and graphs. Students will learn to customize plots for better readability. - Web Scraping
Introduces web scraping using requests and BeautifulSoup. Students will extract and process data from web pages. - API Integration
Covers how to send requests and process JSON responses using APIs. Learners will connect to external services via API calls. - Database Connectivity
Teaches how to connect to SQLite databases and execute SQL queries using Python. Students will create simple CRUD operations. - Capstone Project
Students will apply all the learned concepts in a final project. This will involve building a complete Python application.
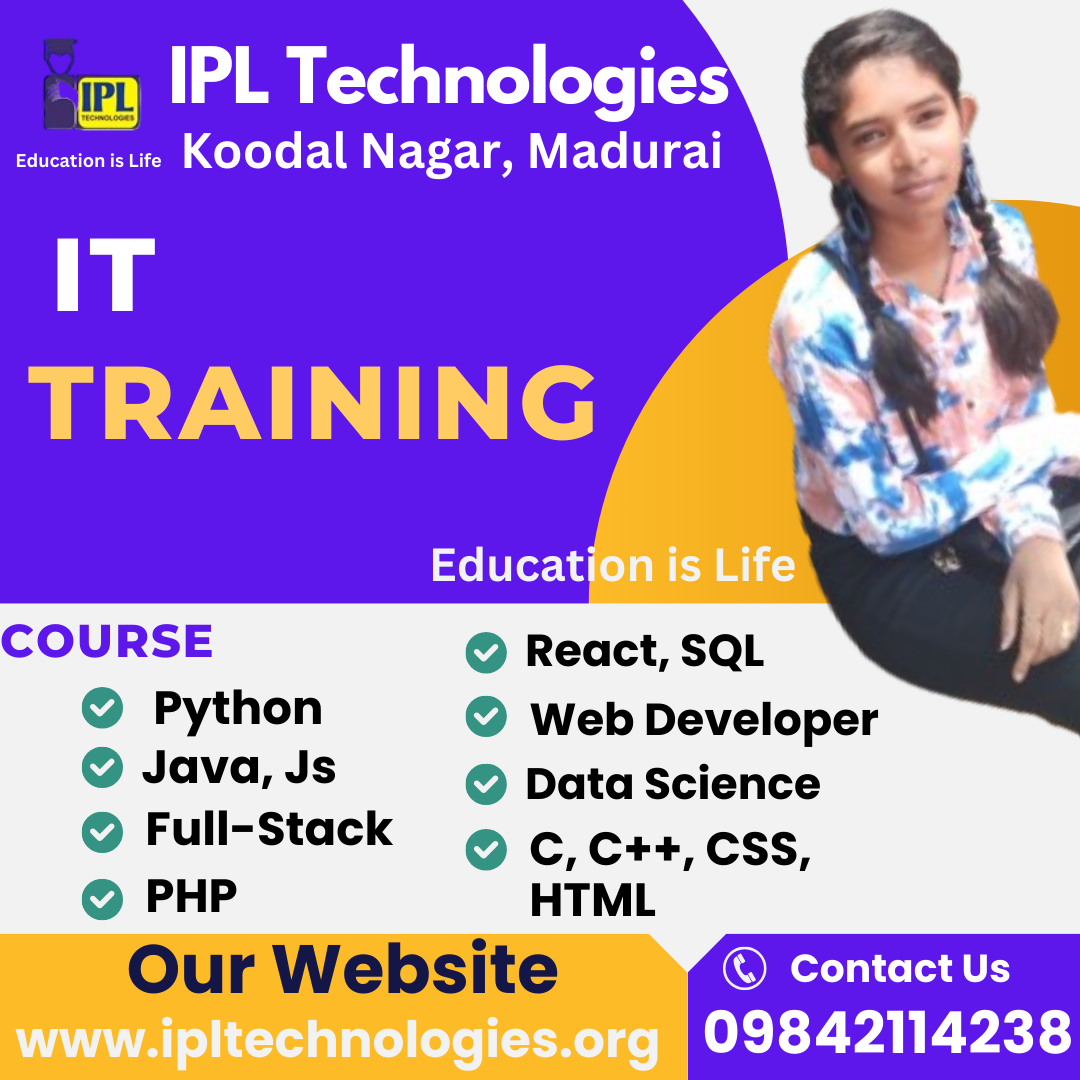