PHP Syllabus
Course Duration: 120 Hrs
- Introduction to PHP
Covers the basics of PHP, its server-side nature, and how it integrates with HTML to build dynamic websites. - Setting up the PHP Environment
Teaches how to install XAMPP/WAMP or LAMP for PHP development and configure the server. - PHP Syntax and Structure
Explains basic syntax, embedding PHP in HTML, and the role of semicolons and case sensitivity. - Variables and Data Types
Covers variable declaration and data types such as integers, strings, floats, and booleans in PHP. - Constants in PHP
Teaches how to define and use constants for fixed values across the application. - Operators in PHP
Explains arithmetic, comparison, logical, and assignment operators with usage examples. - Control Structures
Covers conditional statements (if, else, switch) and loops (for, while, foreach) for logic control. - PHP Functions
Teaches how to define reusable functions, pass parameters, and use return statements. - String Handling in PHP
Explores common string functions like strlen(), str_replace(), and string concatenation. - Arrays and Array Functions
Covers array creation, manipulation, and built-in array functions like array_merge() and sort(). - Associative Arrays and Multidimensional Arrays
Teaches how to create and manipulate associative and nested arrays. - Superglobals in PHP
Explains the role of superglobal variables like $_GET, $_POST, and $_SESSION. - Handling Forms with PHP
Teaches how to process HTML forms using the GET and POST - Session and Cookie Management
Explains how to manage sessions and cookies to maintain user data across pages. - Error Handling in PHP
Covers handling runtime errors using try-catch blocks and custom error handlers. - PHP File Handling
Teaches how to read, write, and append to files using PHP’s file-handling functions. - Working with Dates and Time
Explains how to manage date and time data using PHP functions like date() and time(). - PHP and MySQL Integration
Teaches how to connect PHP applications to MySQL databases for dynamic content management. - CRUD Operations with PHP and MySQL
Covers creating, reading, updating, and deleting data using PHP and MySQL queries. - Prepared Statements and Security
Explains how to use prepared statements to prevent SQL injection attacks. - PHP Object-Oriented Programming (OOP)
Teaches how to create classes, objects, and use OOP concepts like inheritance and polymorphism. - File Uploads in PHP
Covers how to build forms for file uploads and handle the uploaded files on the server. - Working with JSON in PHP
Explains how to encode and decode JSON data for API interactions. - PHP and AJAX Integration
Teaches how to use AJAX with PHP for asynchronous data updates. - PHP and RESTful API Development
Covers creating RESTful APIs in PHP and handling HTTP requests. - Authentication and Authorization
Explains how to implement user login systems with role-based access control. - Email Handling with PHP
Teaches how to send emails using PHP’s mail() function or external SMTP servers. - Working with PHP Frameworks (Laravel)
Introduces Laravel framework for rapid development and best practices in PHP projects. - PHP Unit Testing
Explains the basics of testing PHP code with PHPUnit for code quality assurance. - Using Composer for Dependency Management
Teaches how to use Composer for managing external libraries and packages in PHP projects. - PHP Security Best Practices
Covers secure coding practices, such as input validation, data sanitization, and protection against common vulnerabilities. - Performance Optimization in PHP
Explains techniques for optimizing PHP code and caching strategies for faster applications.
Capstone Project
Students will build a complete web application, applying all the PHP concepts learned throughout the course.
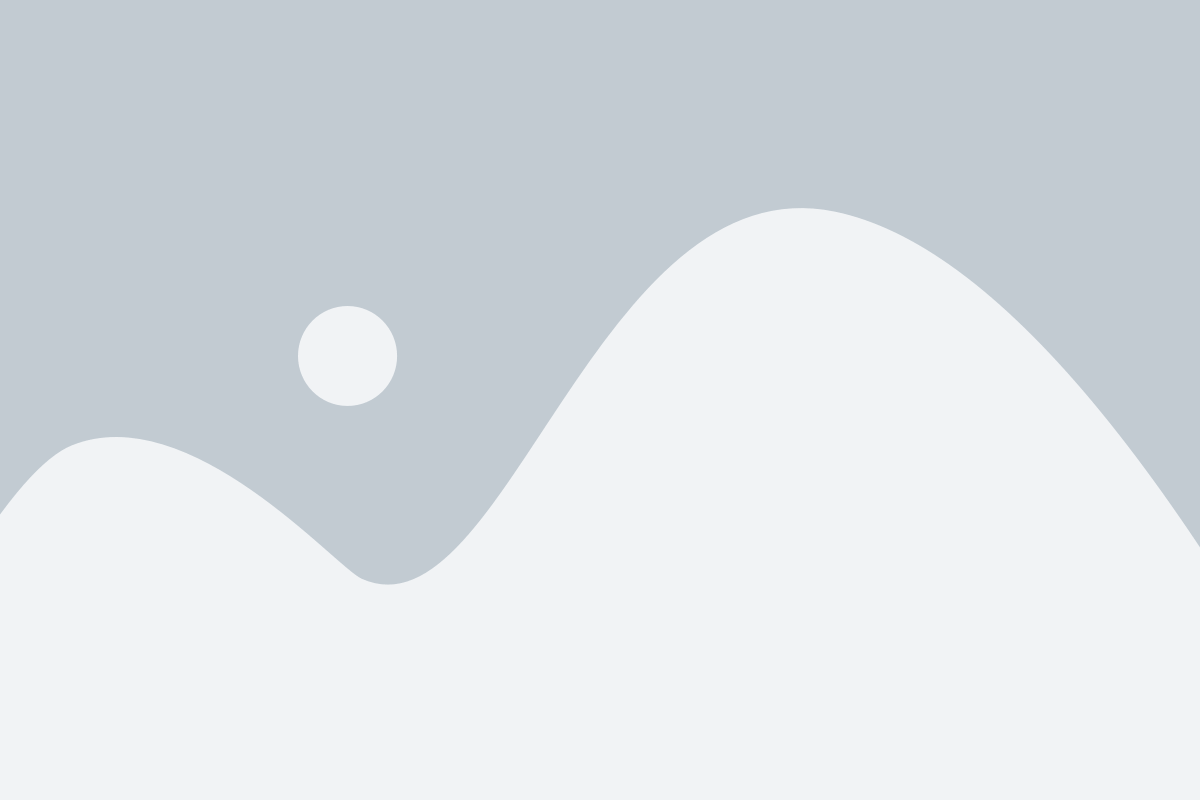